Running queries in a Browser application requires appropriate adjustments in the M.App Editor. You will have several options to retrieve dataset information through a query, which will be detailed in this tutorial.
The real magic happens in the M.App Editor, where you can access and seamlessly integrate a ready-to-use code snippet that allows to execute your query.
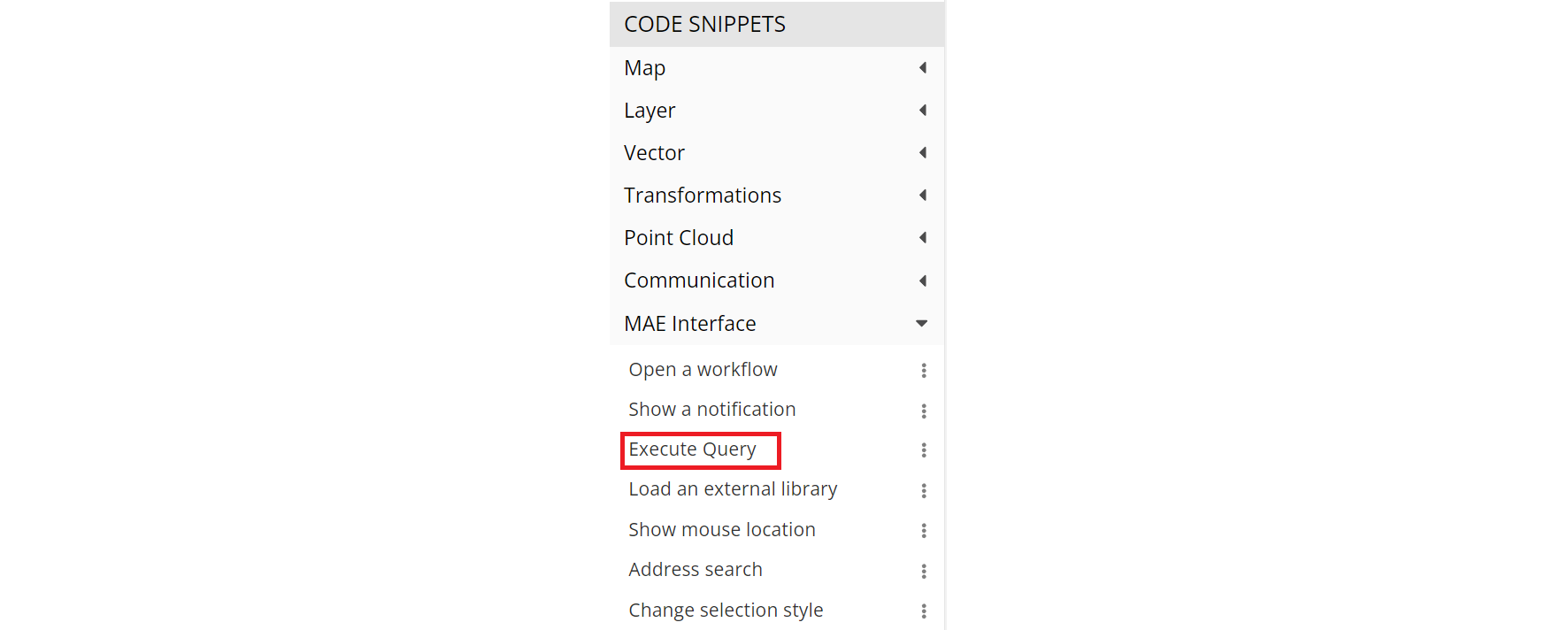
const queryName = 'Query_Name';
const queryRequest = {
format: 'json',
parameters: {},
srid: 3857
}
executeQuery(queryName, queryRequest).then(result => {
console.log(result)
});
Let's have a deeper look into the code snippet. The executeQuery
function is the primary way to run queries on the M.App Enterprise platform. The function varies based on the data format
you request.
- JSON
- CSV
- GeoJSON
The different functions will be elaborated on in the following chapters.
1. JSON Query Execution
declare function executeQuery(queryName: string, queryRequest: QueryJsonRequest): Promise<JsonQueryResponse>;
Two parameters are passed in this function and a promise is returned:
-
queryName
: A string representing the name of the query you want to run. So, basically the name of a query you have created in Studio. queryJsonRequest
: This structure is used when making a request for a JSON format query.Properties of the request:
format
: Always 'json' for this request type, indicating that the result should be in JSON format.parameters
: An object containing the parameters for the query. These are the inputs that the query needs to execute, such as filters or criteria.srid
: A spatial reference ID, which defines the coordinate system for geographic data. If not applicable, this can be null.
JsonQueryResponse
: This is the structure of the response when a query is executed in JSON format and it contains two properties.Properties of the repsonse:
rowCount
: A number indicating how many rows were returned by the query.rows
: An array of data rows, with each row containing the query result data. The type of the rows can be specified if using the generic version.
2. GeoJson Query Execution
declare function executeQuery(queryName: string, queryRequest: QueryGeoJsonRequest): Promise<GeoJsonQueryResponse>;
Two parameters are passed once more, and a promise is returned. Refer to the JSON Query Execution for a detailed explanation of all parameters and properties. Only the differences will be highlighted here.
-
queryName
: same as in Json query queryGeoJsonRequest
: This structure is used when making a request for a GeoJSON format query.Properties of the request:
format
: Always 'geojson' for this request type, indicating that the result should be in GeoJSON format.parameters
: same as in Json querysrid
: same as in Json query
GeoJsonQueryResponse
: This is the structure of the response when a query is executed in GeoJSON format and it contains a number of properties.Properties of the repsonse:
type
: Always 'FeatureCollection', which is a standard type in GeoJSON indicating a collection of features.features
: An array of GeoJsonFeature objects, each representing a geographic feature returned by the query.
Properties of a feature: Each feature in the features array will have further properties which are more specific.
id
: A unique identifier for the feature.type
: Always 'Feature', indicating that this is a GeoJSON feature object.geometry
: An object describing the geometric shape of the feature. The geometry property comes with further specification.
type
: The type of geometric shape such as Point, LineString, Polygon, etc.
coordinates
: The coordinates that define the shape. These can be a single point or an array of points, depending on the type of geometry.properties
: A dictionary of additional attributes or data associated with the feature.
3. CSV Query Execution
declare function executeQuery(queryName: string, queryRequest: QueryCSVRequest): Promise<string>;
Refer to the JSON Query Execution for a detailed explanation of all parameters and properties. Only the differences will be highlighted here.
-
queryName
: same as in JSON query queryCSVRequest
: This structure is used when making a request for a CSV format query.Properties of the request:
format
: Always 'csv' for this request type, indicating that the result should be in CSV format.parameters
: same as in JSON querysrid
: same as in JSON query
string
.
4. Generic Query Execution
declare function executeQuery<T>(queryName: string, queryRequest: QueryJsonRequest): Promisee<JsonQueryResponse<T>>;
Finally, a generic query execution function can be used. This variation of the function allows you to specify the expected data structure.
The query parameters are the same as for JSON query execution, but the returned promise differs.
JsonQueryResponse<T>
:It returns a promise that resolves to a JSON response, but this time with the rows typed according to the type T.